简介
p5从车道检测升级为车辆检测,课程目的是检测行驶过程中遇到的车辆,属于目标检测范畴。这篇文章主要介绍如何使用传统计算机视觉方法进行车辆识别,比较基础。当然实现车辆识别的方案还有其他高大的方案,如yolo等,后面我会开另一篇文章介绍基于深度学习的车辆检测方案。
处理流程
本节课程处理流程如下图所示
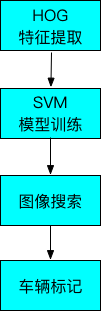
HOG特征提取
梯度直方图(Histogram of Oriented Gradient),简称HOG,它是一种特征描述符。其原理是把图像分成很多个部分,然后计算每个部分的梯度,HOG特征描述符能为特征匹配和目标检测提供非常重要的信息。
为了判断是否是车辆,我们需要分别提取车辆的HOG和非车辆物体的HOG。这里我们使用skimage的hog方法进行图像的特征提取,经过特征提取后的效果如下
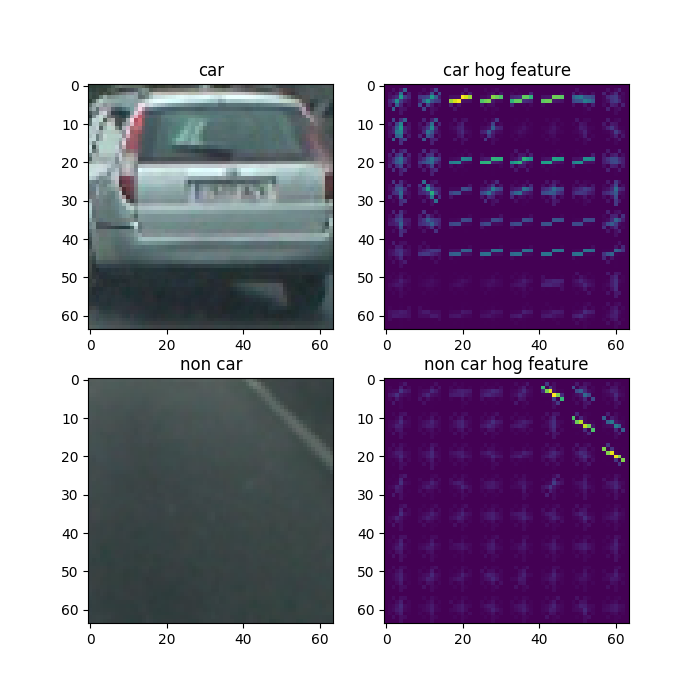
贴上这部分代码
1 | import cv2 |
svm模型训练
我们刚刚获取了车辆和非车辆的hog特征,接下来用这些特征去训练svm模型。
1 | def split_data(): |
图像搜索
接下来进行车辆搜索,其原理是使用滑动窗口截取图像部分区域,然后用刚刚训练好的svm模型判断是否是车辆,如果是就保存该区域坐标。
定义滑动窗口
首先定义滑动窗口,其中有这样几个概念cell、block、window,step。cell是抽取hog特征时的最小单位,这里定义为16个像素。block由cell组成,这里定义为2*2个cell。window是滑动窗口大小,定义为64个像素。step是步长,即每次滑动的长度,定义为2个cell。
1 | #x方向上block个数 |
滑动窗口滑动过程如下
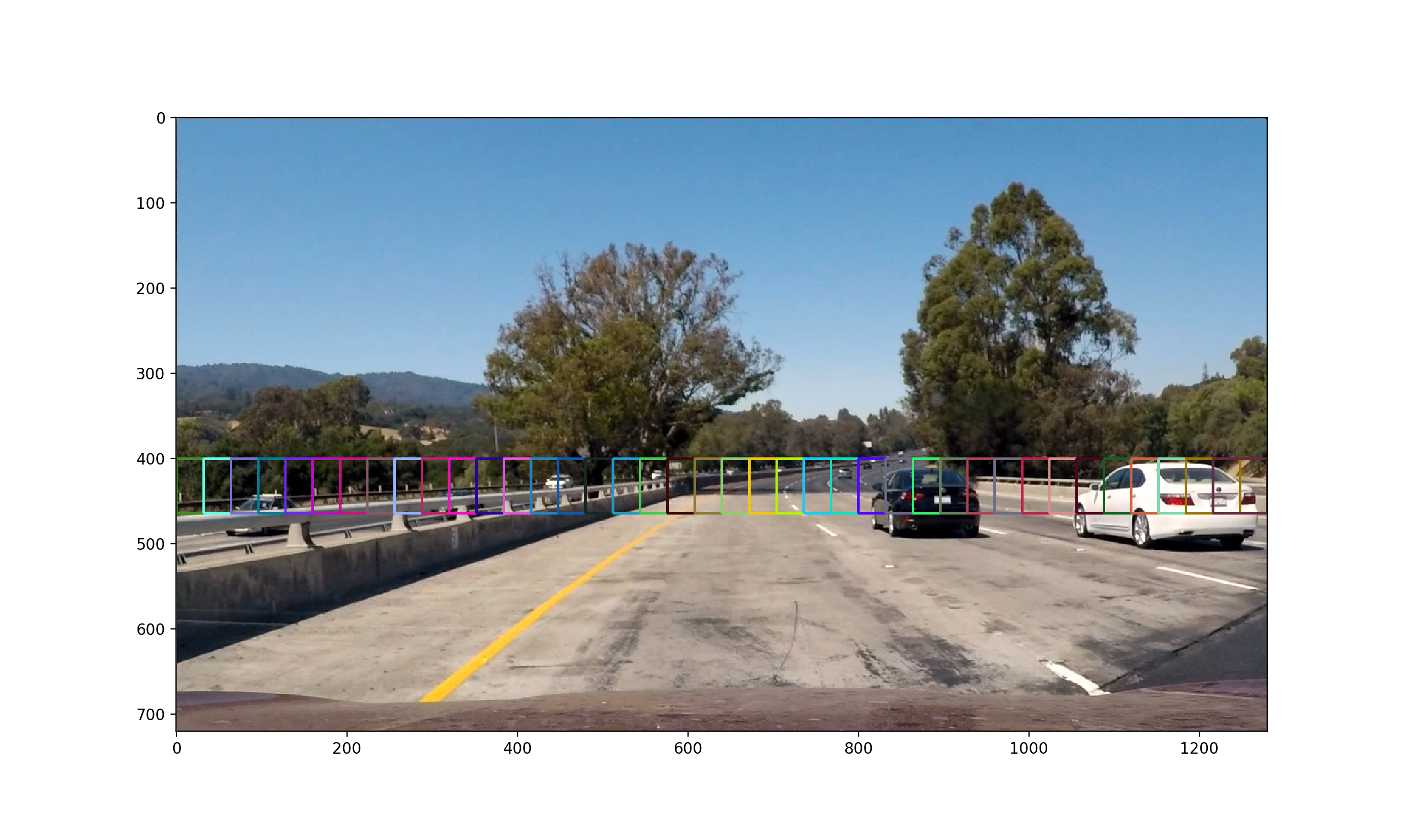
分类
抽取窗口内图像特征,并进行分类,若为车辆,保存其窗口坐标。
1 | #获取特征 |
检测效果
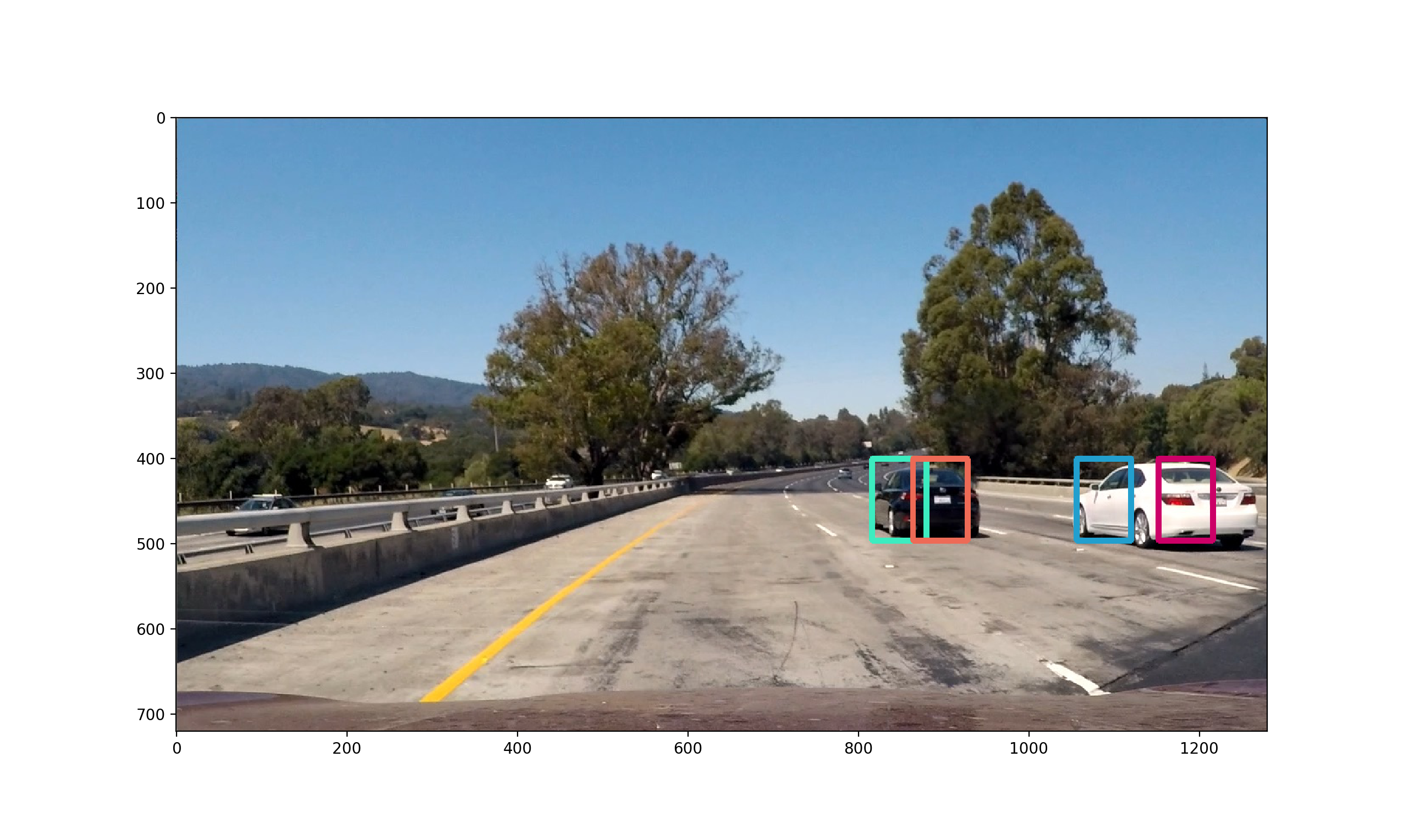
车辆标记
有时候同一辆车可能存在于几个窗口中,需要将其合并,这个过程与非最大抑制类似。我们这里使用heatmap的方式合并窗口,即搜索到车辆的窗口内的像素点计数加1,设置一个阈值,最后只取大于阈值范围像素点构成的窗口。
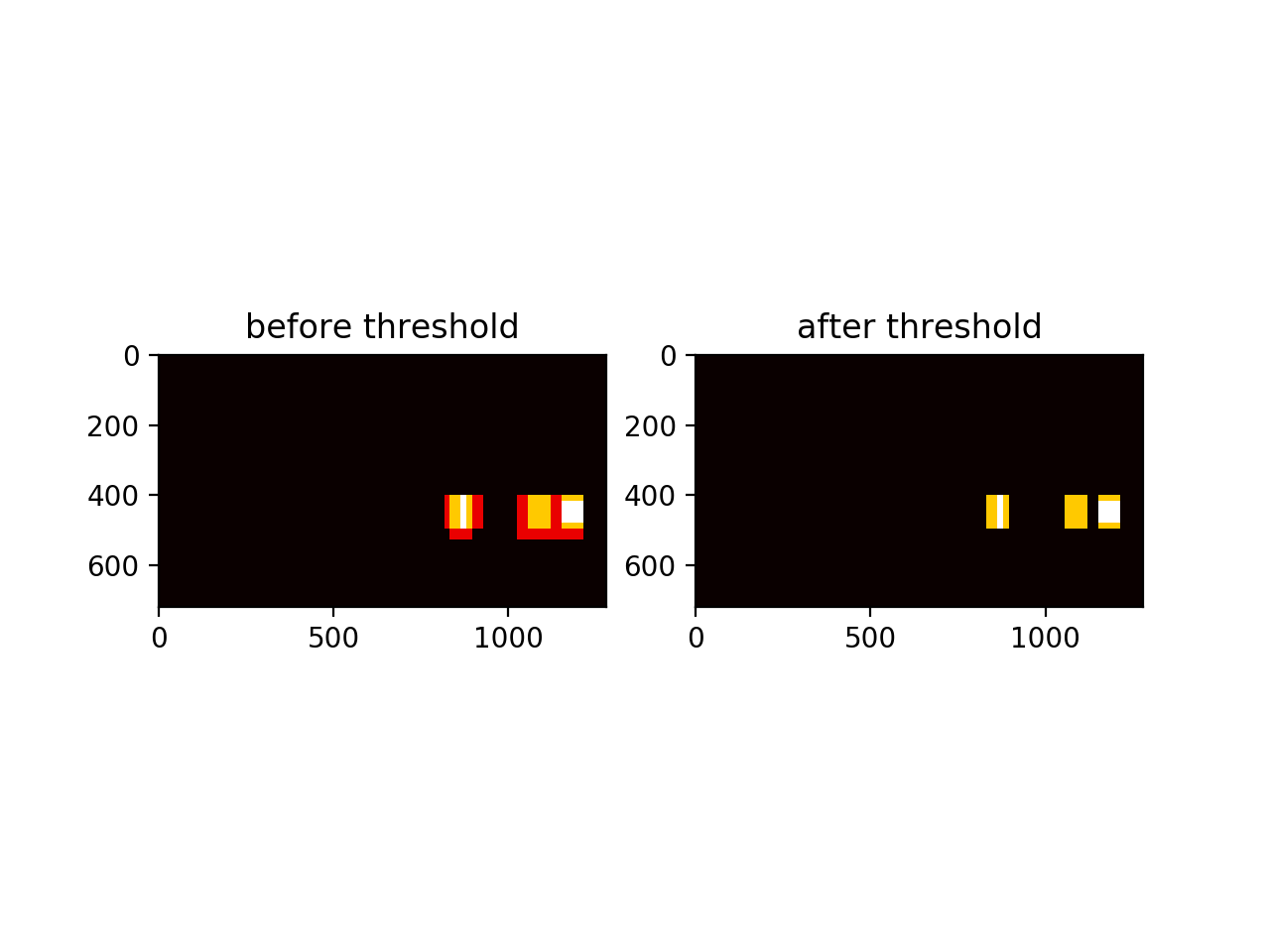
处理视频
原始视频
处理后视频
总结
本节课程主要使用传统计算机视觉方法进行目标检测,其中使用到的技术与常见滑动窗口、图像金字塔和非最大抑制很相似。除了传统计算机视觉方法,还可以使用深度学习进行目标检测,后续再单独开文章进行介绍。这部分代码详见p5-vehicle-detection